Facebook, node.js and Heroku
I have been playing with node.js for a while now. It is a lot of fun but so far it has been all for pleasure - not for work. But now I have finally built my first real, paid project using node.js. And I did it using Heroku to boot. Here are some of the things I learned.
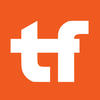
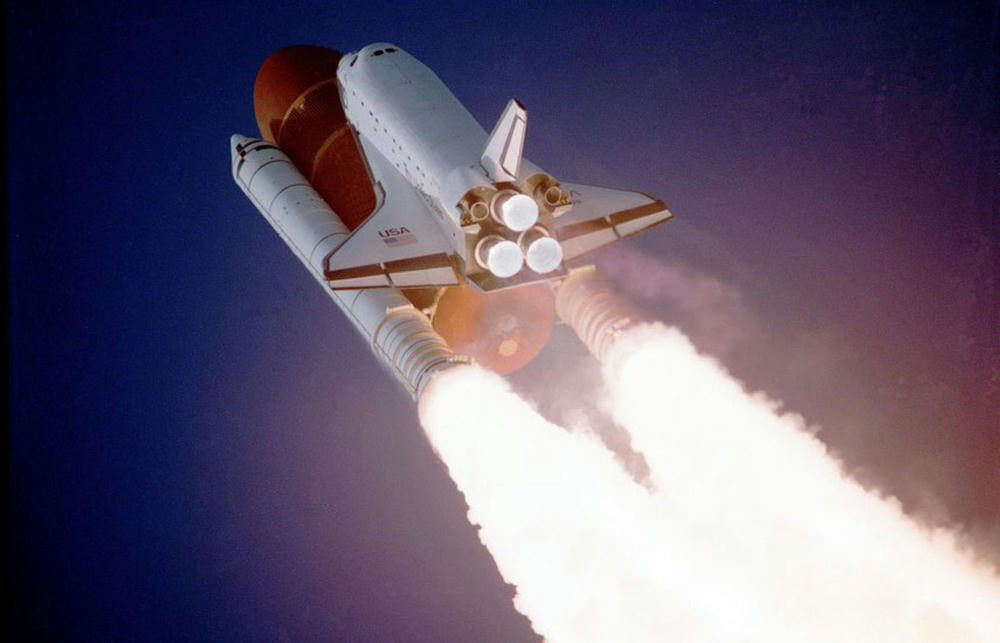
Initial steps
Heroku is a cloud based hosting platform. All you do is register, create an application, add the services you want and push it with Git. Getting started with Heroku for a Facebook app is actually really easy. When creating a Facebook app, you get the ption of hosting it on Heroku. If you choose to do so, Facebook will automagically create an app for you and add some example code. This is where it get's complicated though... at least if you don't know how to proceed.
Testing
This will be your first challenge. You will want to test your code both locally and on Heroku. But setting up two different applications on Facebook takes time. And keeping them in sync during the development process is quite a challenge. Especially during initial development.
What I did was this: I used the fact that Facebook requires a regular AND a secure URL for the app. Heroku provides Piggyback SSL (https://your-app.herokuapp.com) out of the box. So I simply pointed the secure URL at Heroku and the regular URL at localhost. That way, I could switch between the environments by just changing the protocol in the address bar.
Different variables locally and live
A lot of the time, you will want to use different variable values locally/on test and on live. Fortunately Heroku uses Foreman to run its applications. So all you have to do is create an .env-file in your repo and add it to .gitignore. You can now add all your local environment variables to this file like this:
To add your live variable values to Heroku, simply run this in your terminal:
Using socket.io on Heroku
In the application, we used now.js to communicate between the client and the server. Now.js uses socket.io to expose functions between client and server. Heroku uses the Cedar stack. This does not support WebSockets. This means that you will have to restrict socket.io to only use long polling. This is set in the initialization of now:
This will work just fine on http. But not on https. The reason is that now.js adds a socket.io.js script tag and points it at your server including port number. Since Heroku uses the same app for http and https, the port will be set to 80... which obviously doesn't work on https. So you would then set the port number as well, using nothing as the port since this will change between http and https:
But that won't work because now.js uses :80 as a fallback if your port number evaluates to false... which empty string does. Then you try to detect the current port... fail! Then you try to detect the current protocol... fail!
So I came up with this:
...so if I am not running locally (on port 5000), I set the port to an object with a toString function which returns ''. This means that the port does not evaluate to false but it will still produce an empty string when concatenated by now.js.
Other useful stuff
I used quite a lot of packages when building the applications. Here are some of my favourites:
- Now.js (npm install now) for real-time client-server communication
- mongoose (npm install mongoose) for talking to Mongo DB
- fbgraph (npm install fbgraph) for interacting with Facebook's Graph API
- request (npm install request) for making simple http gets
- async (npm install async) for flow management and avoiding the javascript pyramid of doom And my devDependencies for testing:
- mocha (npm install mocha)
- should (npm install should) Also, to make life simpler, I allways add two scripts to my package.json file:
So to start my application, I just call npm start. And to test it using a fangastic Nyan cat test report, I call npm test.
Happy hacking!